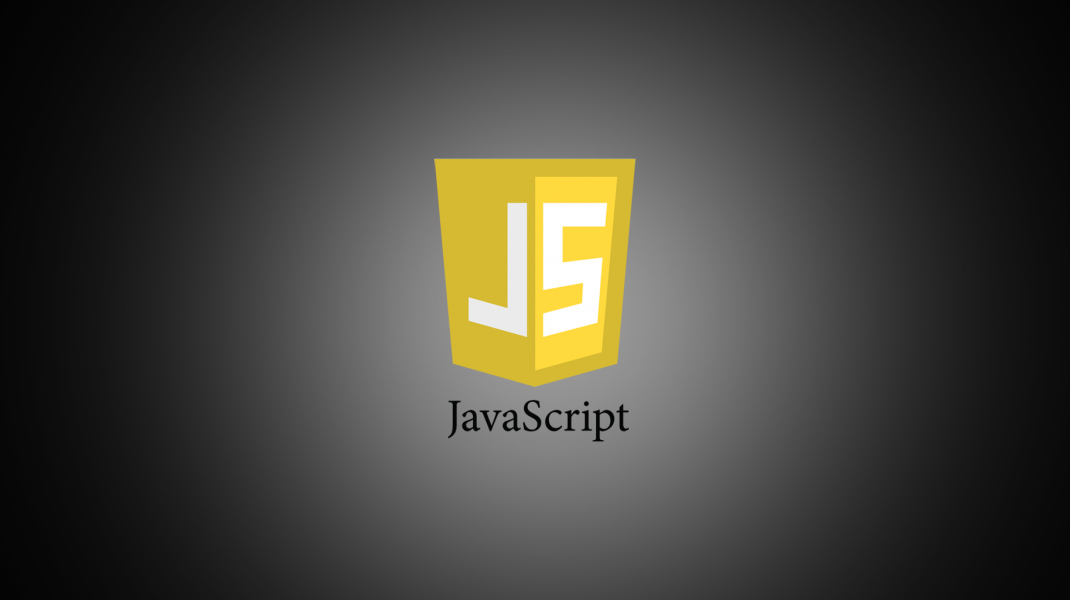
Very Simple Line Graph With D3
I’ve been learning more about D3 and decided to apply what I learned about composition to make a very simple line graph. I’ll just post the pen and comment on it below: See the Pen Very Simple Line Graph with D3 by Mike Newell (@newshorts) on CodePen. Basically, the important parts are that I’m using a factory to create “LineGraph()”.